Published
- 3 min read
Sending mails with domain email smtp server - Golang
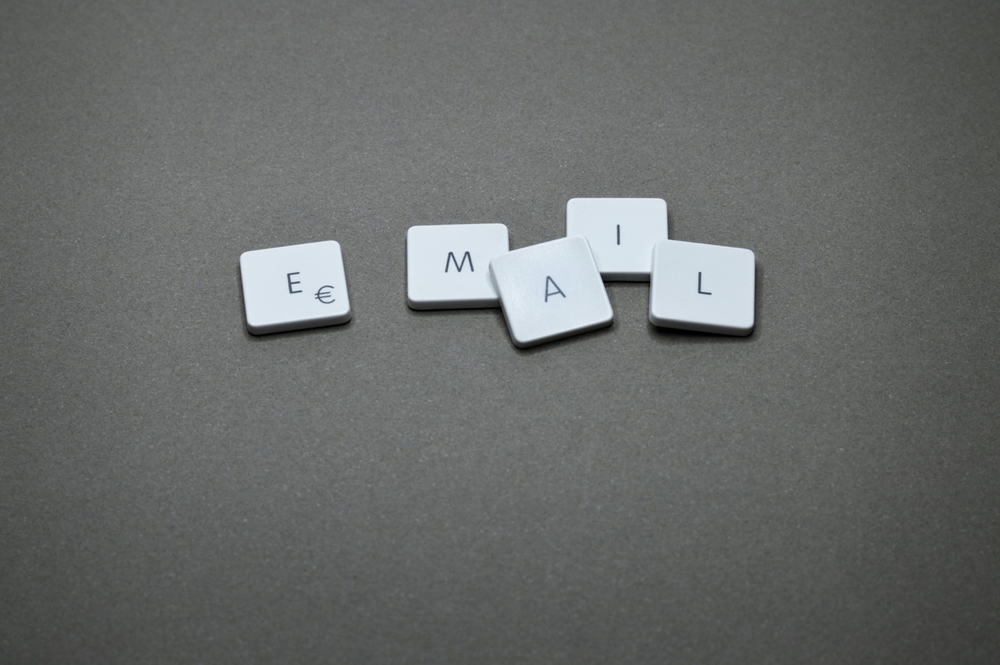
Sending emails is really important to every SaaS I would say. Usually, this is done with email delivery services like SendGrid, MailGun, Amazon SES, etc. These services work great and are perfect for certain use cases but this article is focused on developers needing to work with something free for their side projects or pet projects that do not bring in revenue. I mean it makes sense to not have to pay for a service if you would be underutilizing it. Mailgun for instance, has its basic tier at 10,000 mails per month as at the time of writing this. I definitely do not need to send that many for my personal project or an opensource tool with less than a thousand users.
“The best things in life are free”
- No one* - you can trust this though, no one would be held responsible.*
I will be the using the Gin network library, the gomail package (https://github.com/go-gomail/gomail) and the godotenv package for this. The gomail package is a simple and efficient package to send emails with Go using an smtp server while the godotenv package helps to load env files into the environment.
Using smtp server of email hosting services
If you purchased your domain address from a domain registrar like Namecheap, GoDaddy, BlueHost, etc, they all typically have an associated email hosting service. I use Namecheap and the associated email hosting for namecheap is Privateemail. But the concept is typically the same for them.
The first step is to get the app password (if gmail or google workspace) or password of your domain email address (e.g. hey@example.com) and add it to your env
.env file
MAIL_PASSWORD=abcdefgh123!!!
![]()
![]()
Using the gomail package, the next step is to write a sample function that sends email to an hardcoded email address. You can always pass in an email address to it. This is just for the purpose of demonstration.
func SendEmail() error { err := godotenv.Load(".env") if err != nil { fmt.Println("Error loading .env file ", err) return err } m := gomail.NewMessage() m.SetHeader("From", "Gomail<hey@example.com>") m.SetHeader("To", "example@email.com") m.SetHeader("Subject", "Hello there") m.SetBody("text/plain", "Are you getting this") d := gomail.NewDialer("mail.privateemail.com", 465, "hey@example.com", os.Getenv("MAIL_PASSWORD")) if err := d.DialAndSend(m); err != nil { fmt.Println("Failed to send email", err) return err } fmt.Println("Email Sent Successfully!") return nil }
![]()
![]()
Below is the main function that declares a post request to send the email\
import ( "fmt" "net/http" "os" "github.com/gin-gonic/gin" "github.com/joho/godotenv" "gopkg.in/gomail.v2" ) func main() { server:= gin.Default() server.POST("/sendEmail", func(ctx * gin.Context) { if err := SendEmail(); err != nil { fmt.Println("error ", err.Error()) ctx.JSON(http.StatusBadRequest, gin.H{ "error": "Error sending email" }) return } ctx.JSON(http.StatusOK, gin.H{ "message": "Email send successfully" }) }) server.Run() }
![]()
![]()
Making a post request to /sendEmail should send the email to the receipient
Conclusion
Like earlier stated, this is a quick and free way to send emails without integrating with a third party service at a fee. WIth these smtp servers, you typically get like 500 emails/day which is more than enough for usecases stated earlier. Google workspace smtp server gives you up to 2000 mails/day.
Though this is focused on Golang, there are also ways to do this in other backend stacks.